ok, we are going to play with a little RFID…. I have messed a lot with RFID access cards, IOProx, HID, etc…. but not much with the EM4100 and EM4305 so that is what I am playing with right now. I will probably write some notes up on HID and IOProx later….
Parts:
– Raspberry Pi Zero W – with SD Card obviously
– RDM6300 module and antenne
– Logic Level Shifter
– jumper wires
Start with a headless install of a Raspberry Pi – like from these notes here
Now you need to wire up this stuff…..first the pinouts
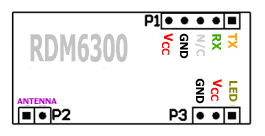
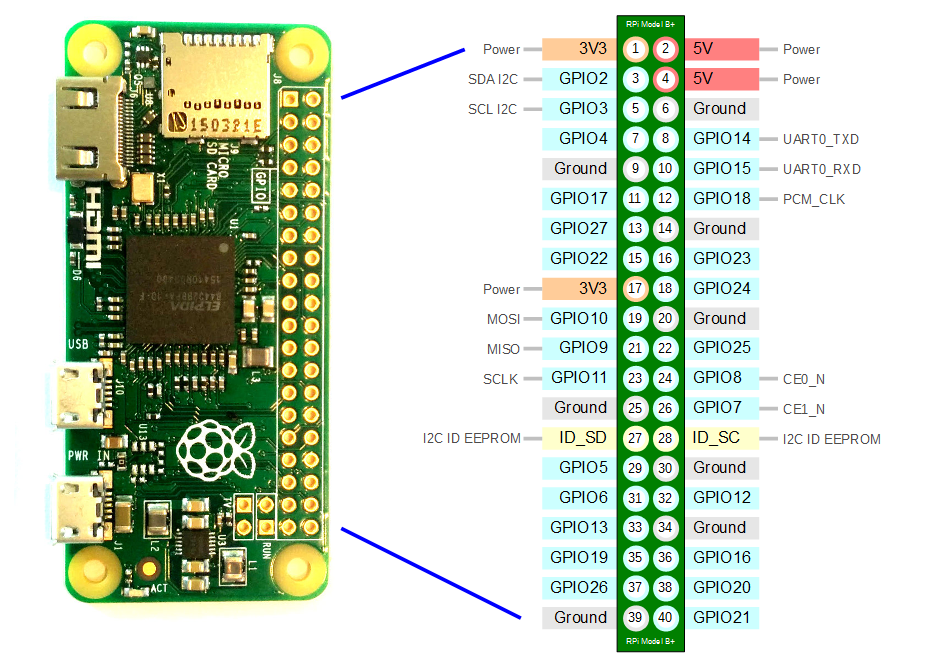
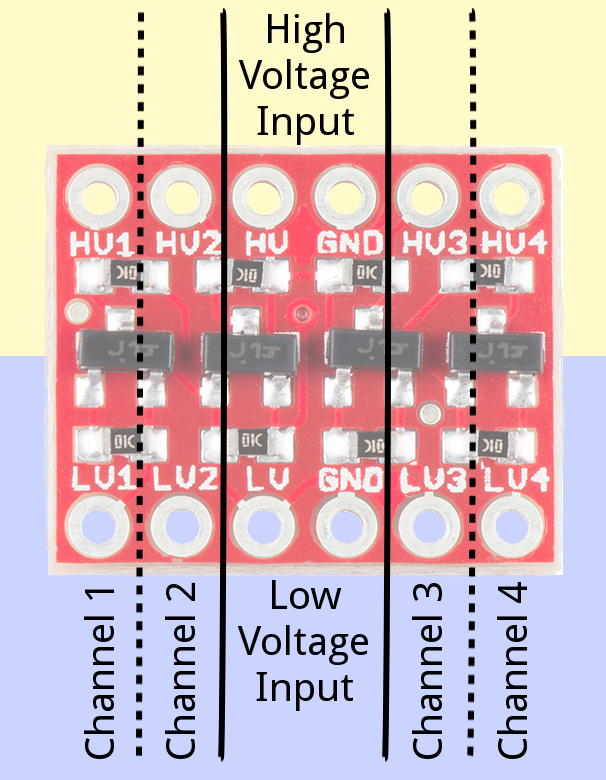
Now we gotta wire it all together…here is my poor attempt at portraying that…. I did use this page for some help, but it is a little old – here.
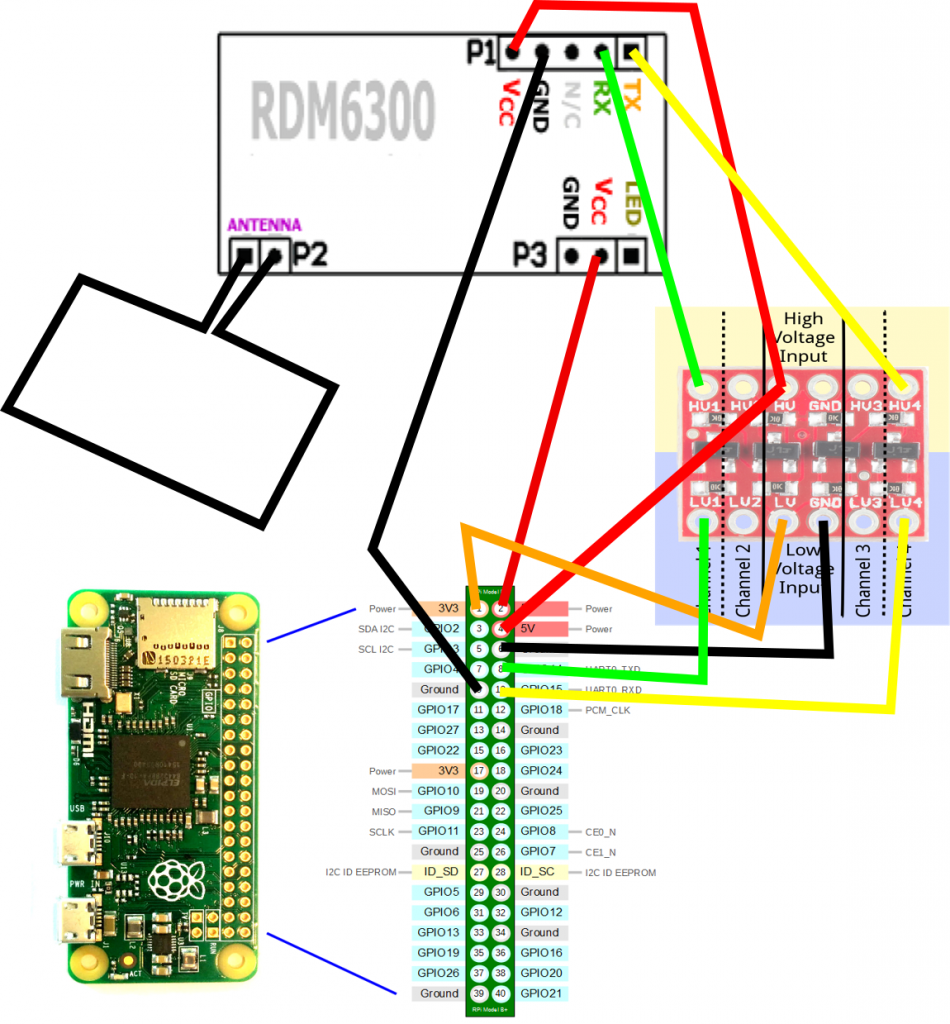
You now need to enable/disable some stuff on the regular raspian image….first run “sudo raspi-config”
– Select “Interfacing Options” this was number 5 for me
– Now select “Serial” which was A7 I think….for me
– Now disable it so select “No”
You can exit out of the raspi-config now. Next we need to enable uart=1 in the /boot/config.txt file, it is usually commented out or set to uart=0 at about the bottom of the file, for me it was the last line and I just modified it to be “uart=1”
REBOOT it! just to be sure your settings went into affect.
ok, now I am working on the code…which I did take from that link above and have expanded it. The key thing of note is to change the serial port to be /dev/serial0 as opposed to the /dev/ttyAMA0 since now that is linked to some other device now…. I named the file RFID.py
import time
import serial
import RPi.GPIO as GPIO
GPIO.setmode(GPIO.BCM)
Tag1 = str('0000000C000C')
Tag2 = str('0000000C080C')
Tag3 = str('0000000C010C')
Tag4 = str('0000000C090C')
Tag5 = str('0000000C0A0C')
Tag6 = str('0000000C0D0C')
GPIO.setup(23,GPIO.OUT)
GPIO.setup(24,GPIO.OUT)
GPIO.output(23,False)
GPIO.output(24,False)
PortRF = serial.Serial('/dev/serial0',9600)
PortRF.reset_input_buffer()
while True:
ID = ""
read_byte = PortRF.read()
if read_byte=="\x02":
for Counter in range(12):
read_byte=PortRF.read()
ID = ID + str(read_byte)
print hex(ord( read_byte))
print ID
if ID == Tag1:
print "White - Ashoka"
GPIO.output(23,True)
GPIO.output(24,False)
PortRF.reset_input_buffer()
time.sleep(5)
GPIO.output(23,False)
elif ID == Tag2:
print "White - Chirutt"
GPIO.output(23,True)
GPIO.output(24,False)
PortRF.reset_input_buffer()
time.sleep(5)
GPIO.output(23,False)
elif ID == Tag3:
print "Red - Vader"
GPIO.output(23,True)
GPIO.output(24,False)
PortRF.reset_input_buffer()
time.sleep(5)
GPIO.output(23,False)
else:
GPIO.output(23,False)
print "Access Denied"
GPIO.output(24,True)
PortRF.reset_input_buffer()
time.sleep(5)
GPIO.output(24,False)
I just run it with “python RFID.py” and when I put one of my EM4100 or EM4305 cards/fobs/objects it will read it and display the rfid number….now time to expand and make this even better!